使用Express框架在服务端创建API
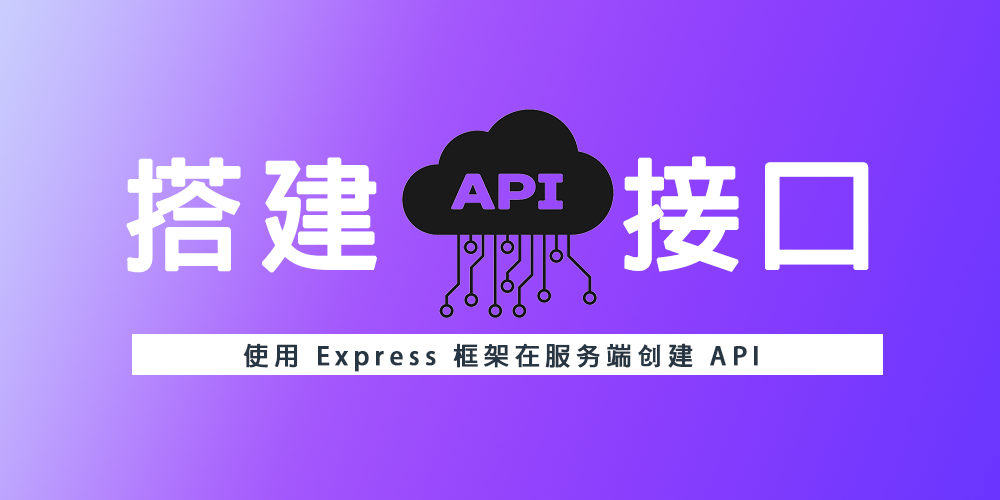
使用Express框架在服务端创建API
乐活星球环境准备
确保服务器端已经安装了Node.js和npm。
初始化项目
创建一个新的项目文件夹,并在该文件夹中运行以下命令来初始化项目:
1 | npm init -y |
安装Express
1 | npm install express |
添加代码
创建一个名为 index.js 的文件,并添加以下代码:
1 | const express = require('express'); |
启动服务
在项目文件夹中运行以下命令来启动服务器:
1 | node index.js |
现在,你的API服务器应该在 http://localhost:3000 上运行。你可以通过浏览器访问该地址,或者使用工具(如Postman)来测试POST请求。
安装 PM2
使用 npm(Node.js 的包管理器)全局安装 PM2:
1 | sudo npm install pm2@latest -g |
验证安装
安装完成后,可以通过以下命令验证 PM2 是否安装成功,如果输出显示 PM2 的版本号,则表示 PM2 已成功安装。
1 | pm2 -v |
启动应用程序
使用 PM2 启动你的应用程序,例如 index.js:
1 | node index.js |
查看应用程序状态
查看所有运行中的应用程序及其状态:
1 | pm2 list |
设置开机自启
配置 PM2 在服务器重启时自动启动应用程序:
1 | pm2 startup |
使用pm2重启所有程序
1 | pm2 restart all |
评论
匿名评论隐私政策
✅ 你无需删除空行,直接评论以获取最佳展示效果